mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 22:00:47 +01:00
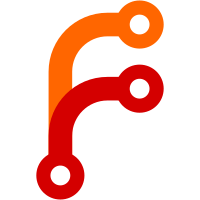
This is a safe cleanup change since top-level const applied to parameters in function declarations (that are not also definitions) are ignored by the compiler. Hence, such changes do not change the type of the declared functions and are simply no-ops. Bug: webrtc:13610 Change-Id: Ibafb92c45119a6d8bdb6f9109aa8dad6385163a9 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/249086 Reviewed-by: Niels Moller <nisse@webrtc.org> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Commit-Queue: Ali Tofigh <alito@webrtc.org> Cr-Commit-Position: refs/heads/main@{#35802}
60 lines
2.3 KiB
C++
60 lines
2.3 KiB
C++
/*
|
|
* Copyright (c) 2013 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_AUDIO_CODING_NETEQ_TOOLS_INPUT_AUDIO_FILE_H_
|
|
#define MODULES_AUDIO_CODING_NETEQ_TOOLS_INPUT_AUDIO_FILE_H_
|
|
|
|
#include <stdio.h>
|
|
|
|
#include <string>
|
|
|
|
namespace webrtc {
|
|
namespace test {
|
|
|
|
// Class for handling a looping input audio file.
|
|
class InputAudioFile {
|
|
public:
|
|
explicit InputAudioFile(std::string file_name, bool loop_at_end = true);
|
|
|
|
virtual ~InputAudioFile();
|
|
|
|
InputAudioFile(const InputAudioFile&) = delete;
|
|
InputAudioFile& operator=(const InputAudioFile&) = delete;
|
|
|
|
// Reads `samples` elements from source file to `destination`. Returns true
|
|
// if the read was successful, otherwise false. If the file end is reached,
|
|
// the file is rewound and reading continues from the beginning.
|
|
// The output `destination` must have the capacity to hold `samples` elements.
|
|
virtual bool Read(size_t samples, int16_t* destination);
|
|
|
|
// Fast-forwards (`samples` > 0) or -backwards (`samples` < 0) the file by the
|
|
// indicated number of samples. Just like Read(), Seek() starts over at the
|
|
// beginning of the file if the end is reached. However, seeking backwards
|
|
// past the beginning of the file is not possible.
|
|
virtual bool Seek(int samples);
|
|
|
|
// Creates a multi-channel signal from a mono signal. Each sample is repeated
|
|
// `channels` times to create an interleaved multi-channel signal where all
|
|
// channels are identical. The output `destination` must have the capacity to
|
|
// hold samples * channels elements. Note that `source` and `destination` can
|
|
// be the same array (i.e., point to the same address).
|
|
static void DuplicateInterleaved(const int16_t* source,
|
|
size_t samples,
|
|
size_t channels,
|
|
int16_t* destination);
|
|
|
|
private:
|
|
FILE* fp_;
|
|
const bool loop_at_end_;
|
|
};
|
|
|
|
} // namespace test
|
|
} // namespace webrtc
|
|
#endif // MODULES_AUDIO_CODING_NETEQ_TOOLS_INPUT_AUDIO_FILE_H_
|