mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-20 09:07:52 +01:00
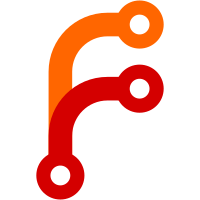
There are two threads involved here, the thread that calls the API functions and the pipwire main loop. Using one race checker for both is wrong and triggers aborts. Use a different race checker for all variables that are used by the pipewire main loop or guarded against concurrent access with the thread_loop_lock. In one case, two RTC_CHECK_RUNS_SERIALIZED() checks are needed, so enhance the macro to generate unique variable names. Bug: webrtc:15181 Change-Id: Ib41514eb7aa98fe85d830461aa0c71e42ba821bd Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/326781 Reviewed-by: Ilya Nikolaevskiy <ilnik@webrtc.org> Commit-Queue: Tomas Gunnarsson <tommi@webrtc.org> Reviewed-by: Tomas Gunnarsson <tommi@webrtc.org> Cr-Commit-Position: refs/heads/main@{#41198}
61 lines
2.3 KiB
C++
61 lines
2.3 KiB
C++
/*
|
|
* Copyright (c) 2022 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_VIDEO_CAPTURE_LINUX_VIDEO_CAPTURE_PIPEWIRE_H_
|
|
#define MODULES_VIDEO_CAPTURE_LINUX_VIDEO_CAPTURE_PIPEWIRE_H_
|
|
|
|
#include "modules/video_capture/linux/pipewire_session.h"
|
|
#include "modules/video_capture/video_capture_defines.h"
|
|
#include "modules/video_capture/video_capture_impl.h"
|
|
|
|
namespace webrtc {
|
|
namespace videocapturemodule {
|
|
class VideoCaptureModulePipeWire : public VideoCaptureImpl {
|
|
public:
|
|
explicit VideoCaptureModulePipeWire(VideoCaptureOptions* options);
|
|
~VideoCaptureModulePipeWire() override;
|
|
int32_t Init(const char* deviceUniqueId);
|
|
int32_t StartCapture(const VideoCaptureCapability& capability) override;
|
|
int32_t StopCapture() override;
|
|
bool CaptureStarted() override;
|
|
int32_t CaptureSettings(VideoCaptureCapability& settings) override;
|
|
|
|
static VideoType PipeWireRawFormatToVideoType(uint32_t format);
|
|
|
|
private:
|
|
static void OnStreamParamChanged(void* data,
|
|
uint32_t id,
|
|
const struct spa_pod* format);
|
|
static void OnStreamStateChanged(void* data,
|
|
pw_stream_state old_state,
|
|
pw_stream_state state,
|
|
const char* error_message);
|
|
|
|
static void OnStreamProcess(void* data);
|
|
|
|
void OnFormatChanged(const struct spa_pod* format);
|
|
void ProcessBuffers();
|
|
|
|
rtc::RaceChecker pipewire_checker_;
|
|
|
|
const rtc::scoped_refptr<PipeWireSession> session_
|
|
RTC_GUARDED_BY(capture_checker_);
|
|
int node_id_ RTC_GUARDED_BY(capture_checker_);
|
|
VideoCaptureCapability configured_capability_
|
|
RTC_GUARDED_BY(pipewire_checker_);
|
|
bool started_ RTC_GUARDED_BY(api_lock_);
|
|
|
|
struct pw_stream* stream_ RTC_GUARDED_BY(pipewire_checker_) = nullptr;
|
|
struct spa_hook stream_listener_ RTC_GUARDED_BY(pipewire_checker_);
|
|
};
|
|
} // namespace videocapturemodule
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_VIDEO_CAPTURE_LINUX_VIDEO_CAPTURE_PIPEWIRE_H_
|