mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
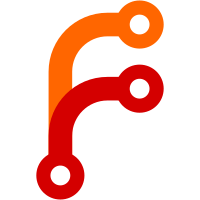
This is mainly a refactoring commit, to break out packet sending to a dedicated component. Bug: webrtc:12943 Change-Id: I78f18933776518caf49737d3952bda97f19ef335 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/228565 Reviewed-by: Florent Castelli <orphis@webrtc.org> Commit-Queue: Victor Boivie <boivie@webrtc.org> Cr-Commit-Position: refs/heads/master@{#34772}
50 lines
1.7 KiB
C++
50 lines
1.7 KiB
C++
/*
|
|
* Copyright (c) 2021 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#include "net/dcsctp/socket/packet_sender.h"
|
|
|
|
#include "net/dcsctp/common/internal_types.h"
|
|
#include "net/dcsctp/packet/chunk/cookie_ack_chunk.h"
|
|
#include "net/dcsctp/socket/mock_dcsctp_socket_callbacks.h"
|
|
#include "rtc_base/gunit.h"
|
|
#include "test/gmock.h"
|
|
|
|
namespace dcsctp {
|
|
namespace {
|
|
using ::testing::_;
|
|
|
|
constexpr VerificationTag kVerificationTag(123);
|
|
|
|
class PacketSenderTest : public testing::Test {
|
|
protected:
|
|
PacketSenderTest() : sender_(callbacks_, on_send_fn_.AsStdFunction()) {}
|
|
|
|
SctpPacket::Builder PacketBuilder() const {
|
|
return SctpPacket::Builder(kVerificationTag, options_);
|
|
}
|
|
|
|
DcSctpOptions options_;
|
|
testing::NiceMock<MockDcSctpSocketCallbacks> callbacks_;
|
|
testing::MockFunction<void(rtc::ArrayView<const uint8_t>, SendPacketStatus)>
|
|
on_send_fn_;
|
|
PacketSender sender_;
|
|
};
|
|
|
|
TEST_F(PacketSenderTest, SendPacketCallsCallback) {
|
|
EXPECT_CALL(on_send_fn_, Call(_, SendPacketStatus::kSuccess));
|
|
EXPECT_TRUE(sender_.Send(PacketBuilder().Add(CookieAckChunk())));
|
|
|
|
EXPECT_CALL(callbacks_, SendPacketWithStatus)
|
|
.WillOnce(testing::Return(SendPacketStatus::kError));
|
|
EXPECT_CALL(on_send_fn_, Call(_, SendPacketStatus::kError));
|
|
EXPECT_FALSE(sender_.Send(PacketBuilder().Add(CookieAckChunk())));
|
|
}
|
|
|
|
} // namespace
|
|
} // namespace dcsctp
|