mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 14:20:45 +01:00
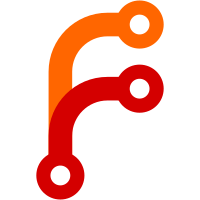
This reverts commit27f3bf5128
. Reason for revert: Broken internal project. Original change's description: > Reland "Replace BundleFilter with RtpDemuxer in RtpTransport." > > This reverts commit97d5e5b32c
. > > Reason for revert: <INSERT REASONING HERE> > > Original change's description: > > Revert "Replace BundleFilter with RtpDemuxer in RtpTransport." > > > > This reverts commitea8b62a3e7
. > > > > Reason for revert: Broke chromium tests. > > Original change's description: > > > Replace BundleFilter with RtpDemuxer in RtpTransport. > > > > > > BundleFilter is replaced by RtpDemuxer in RtpTransport for payload > > > type-based demuxing. RtpTransport will support MID-based demuxing later. > > > > > > Each BaseChannel has its own RTP demuxing criteria and when connecting > > > to the RtpTransport, BaseChannel will register itself as a demuxer sink. > > > > > > The inheritance model is changed. New inheritance chain: > > > DtlsSrtpTransport->SrtpTransport->RtpTranpsort > > > > > > NOTE: > > > When RTCP packets are received, Call::DeliverRtcp will be called for > > > multiple times (webrtc:9035) which is an existing issue. With this CL, > > > it will become more of a problem and should be fixed. > > > > > > Bug: webrtc:8587 > > > Change-Id: I1d8a00443bd4bcbacc56e5e19b7294205cdc38f0 > > > Reviewed-on: https://webrtc-review.googlesource.com/61360 > > > Commit-Queue: Zhi Huang <zhihuang@webrtc.org> > > > Reviewed-by: Steve Anton <steveanton@webrtc.org> > > > Reviewed-by: Taylor Brandstetter <deadbeef@webrtc.org> > > > Cr-Commit-Position: refs/heads/master@{#22613} > > > > TBR=steveanton@webrtc.org,deadbeef@webrtc.org,zhihuang@webrtc.org > > > > Change-Id: If245da9d1ce970ac8dab7f45015e9b268a5dbcbd > > No-Presubmit: true > > No-Tree-Checks: true > > No-Try: true > > Bug: webrtc:8587 > > Reviewed-on: https://webrtc-review.googlesource.com/64860 > > Reviewed-by: Zhi Huang <zhihuang@webrtc.org> > > Commit-Queue: Zhi Huang <zhihuang@webrtc.org> > > Cr-Commit-Position: refs/heads/master@{#22614} > > TBR=steveanton@webrtc.org,deadbeef@webrtc.org,zhihuang@webrtc.org > > Change-Id: I3c272588ab4388ecadc4edc6786d5195c701855f > No-Presubmit: true > No-Tree-Checks: true > No-Try: true > Bug: webrtc:8587 > Reviewed-on: https://webrtc-review.googlesource.com/64862 > Commit-Queue: Zhi Huang <zhihuang@webrtc.org> > Reviewed-by: Zhi Huang <zhihuang@webrtc.org> > Cr-Commit-Position: refs/heads/master@{#22615} TBR=steveanton@webrtc.org,deadbeef@webrtc.org,zhihuang@webrtc.org # Not skipping CQ checks because original CL landed > 1 day ago. Bug: webrtc:8587 Change-Id: I694ce9a039ed52c5961cdc0cba57587bed4cbde4 Reviewed-on: https://webrtc-review.googlesource.com/65381 Reviewed-by: Zhi Huang <zhihuang@webrtc.org> Commit-Queue: Zhi Huang <zhihuang@webrtc.org> Cr-Commit-Position: refs/heads/master@{#22665}
54 lines
1.6 KiB
C++
54 lines
1.6 KiB
C++
/*
|
|
* Copyright 2004 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef PC_BUNDLEFILTER_H_
|
|
#define PC_BUNDLEFILTER_H_
|
|
|
|
#include <stdint.h>
|
|
|
|
#include <set>
|
|
#include <vector>
|
|
|
|
#include "media/base/streamparams.h"
|
|
#include "rtc_base/basictypes.h"
|
|
|
|
namespace cricket {
|
|
|
|
// In case of single RTP session and single transport channel, all session
|
|
// (or media) channels share a common transport channel. Hence they all get
|
|
// SignalReadPacket when packet received on transport channel. This requires
|
|
// cricket::BaseChannel to know all the valid sources, else media channel
|
|
// will decode invalid packets.
|
|
//
|
|
// This class determines whether a packet is destined for cricket::BaseChannel.
|
|
// This is only to be used for RTP packets as RTCP packets are not filtered.
|
|
// For RTP packets, this is decided based on the payload type.
|
|
class BundleFilter {
|
|
public:
|
|
BundleFilter();
|
|
~BundleFilter();
|
|
|
|
// Determines if a RTP packet belongs to valid cricket::BaseChannel.
|
|
bool DemuxPacket(const uint8_t* data, size_t len);
|
|
|
|
// Adds the supported payload type.
|
|
void AddPayloadType(int payload_type);
|
|
|
|
// Public for unittests.
|
|
bool FindPayloadType(int pl_type) const;
|
|
void ClearAllPayloadTypes();
|
|
|
|
private:
|
|
std::set<int> payload_types_;
|
|
};
|
|
|
|
} // namespace cricket
|
|
|
|
#endif // PC_BUNDLEFILTER_H_
|