mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
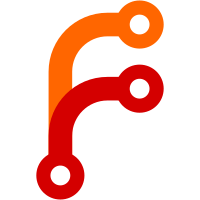
And delete corresponding dependencies on :webrtc_common. After this change, common_types.h is included directly only from code in the following directories: api/ api/video/ api/video_codecs/ common_video/libyuv/include/ media/base/ modules/remote_bitrate_estimator/ modules/rtp_rtcp/source/ modules/video_coding/codecs/vp9/ There remains plenty of indirect dependencies on the types declared in common_types.h, but the fewer direct dependencies should make it easier to find the proper place for each type. Bug: webrtc:5876 Change-Id: I93e8f214025ecb613c19fdec2015bd3f96c59aae Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/130501 Reviewed-by: Karl Wiberg <kwiberg@webrtc.org> Commit-Queue: Niels Moller <nisse@webrtc.org> Cr-Commit-Position: refs/heads/master@{#27376}
61 lines
1.9 KiB
C++
61 lines
1.9 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_VIDEO_CODING_UTILITY_IVF_FILE_WRITER_H_
|
|
#define MODULES_VIDEO_CODING_UTILITY_IVF_FILE_WRITER_H_
|
|
|
|
#include <stddef.h>
|
|
#include <stdint.h>
|
|
#include <memory>
|
|
|
|
#include "api/video/encoded_image.h"
|
|
#include "rtc_base/constructor_magic.h"
|
|
#include "rtc_base/system/file_wrapper.h"
|
|
#include "rtc_base/time_utils.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class IvfFileWriter {
|
|
public:
|
|
// Takes ownership of the file, which will be closed either through
|
|
// Close or ~IvfFileWriter. If writing a frame would take the file above the
|
|
// |byte_limit| the file will be closed, the write (and all future writes)
|
|
// will fail. A |byte_limit| of 0 is equivalent to no limit.
|
|
static std::unique_ptr<IvfFileWriter> Wrap(FileWrapper file,
|
|
size_t byte_limit);
|
|
~IvfFileWriter();
|
|
|
|
bool WriteFrame(const EncodedImage& encoded_image, VideoCodecType codec_type);
|
|
bool Close();
|
|
|
|
private:
|
|
explicit IvfFileWriter(FileWrapper file, size_t byte_limit);
|
|
|
|
bool WriteHeader();
|
|
bool InitFromFirstFrame(const EncodedImage& encoded_image,
|
|
VideoCodecType codec_type);
|
|
|
|
VideoCodecType codec_type_;
|
|
size_t bytes_written_;
|
|
size_t byte_limit_;
|
|
size_t num_frames_;
|
|
uint16_t width_;
|
|
uint16_t height_;
|
|
int64_t last_timestamp_;
|
|
bool using_capture_timestamps_;
|
|
rtc::TimestampWrapAroundHandler wrap_handler_;
|
|
FileWrapper file_;
|
|
|
|
RTC_DISALLOW_COPY_AND_ASSIGN(IvfFileWriter);
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_VIDEO_CODING_UTILITY_IVF_FILE_WRITER_H_
|