mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 14:20:45 +01:00
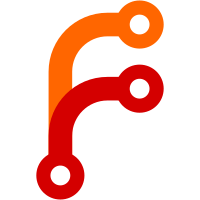
The Chromium implementation unfortunately has a rare deadlock. Rather than patching that up, we're changing the metronome implementation to be able to use a single-threaded environment instead. The metronome functionality is disabled in VideoReceiveStream2 construction inside call.cc. The new design does not have listener registration or deresigstration and instead accepts and invokes callbacks, on the same sequence that requested the callback. This allows the clients to use features such as WeakPtrFactories or ScopedThreadSafety for cancellation. The CL will be followed up with cleanup CLs that removes registration APIs once downstream consumers have adapted. Bug: chromium:1381982 Change-Id: I43732d1971e2276c39b431a04365cd2fc3c55c25 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/282280 Reviewed-by: Per Kjellander <perkj@webrtc.org> Reviewed-by: Erik Språng <sprang@webrtc.org> Reviewed-by: Evan Shrubsole <eshr@webrtc.org> Commit-Queue: Markus Handell <handellm@webrtc.org> Cr-Commit-Position: refs/heads/main@{#38582}
84 lines
2.6 KiB
C++
84 lines
2.6 KiB
C++
/*
|
|
* Copyright 2012 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "pc/test/integration_test_helpers.h"
|
|
|
|
namespace webrtc {
|
|
|
|
PeerConnectionInterface::RTCOfferAnswerOptions IceRestartOfferAnswerOptions() {
|
|
PeerConnectionInterface::RTCOfferAnswerOptions options;
|
|
options.ice_restart = true;
|
|
return options;
|
|
}
|
|
|
|
void RemoveSsrcsAndMsids(cricket::SessionDescription* desc) {
|
|
for (ContentInfo& content : desc->contents()) {
|
|
content.media_description()->mutable_streams().clear();
|
|
}
|
|
desc->set_msid_supported(false);
|
|
desc->set_msid_signaling(0);
|
|
}
|
|
|
|
void RemoveSsrcsAndKeepMsids(cricket::SessionDescription* desc) {
|
|
for (ContentInfo& content : desc->contents()) {
|
|
std::string track_id;
|
|
std::vector<std::string> stream_ids;
|
|
if (!content.media_description()->streams().empty()) {
|
|
const StreamParams& first_stream =
|
|
content.media_description()->streams()[0];
|
|
track_id = first_stream.id;
|
|
stream_ids = first_stream.stream_ids();
|
|
}
|
|
content.media_description()->mutable_streams().clear();
|
|
StreamParams new_stream;
|
|
new_stream.id = track_id;
|
|
new_stream.set_stream_ids(stream_ids);
|
|
content.media_description()->AddStream(new_stream);
|
|
}
|
|
}
|
|
|
|
int FindFirstMediaStatsIndexByKind(
|
|
const std::string& kind,
|
|
const std::vector<const webrtc::DEPRECATED_RTCMediaStreamTrackStats*>&
|
|
media_stats_vec) {
|
|
for (size_t i = 0; i < media_stats_vec.size(); i++) {
|
|
if (media_stats_vec[i]->kind.ValueToString() == kind) {
|
|
return i;
|
|
}
|
|
}
|
|
return -1;
|
|
}
|
|
|
|
TaskQueueMetronome::TaskQueueMetronome(TimeDelta tick_period)
|
|
: tick_period_(tick_period) {}
|
|
|
|
void TaskQueueMetronome::RequestCallOnNextTick(
|
|
absl::AnyInvocable<void() &&> callback) {
|
|
callbacks_.push_back(std::move(callback));
|
|
// Only schedule a tick callback for the first `callback` addition.
|
|
// Schedule on the current task queue to comply with RequestCallOnNextTick
|
|
// requirements.
|
|
if (callbacks_.size() == 1) {
|
|
TaskQueueBase::Current()->PostDelayedTask(
|
|
[this] {
|
|
std::vector<absl::AnyInvocable<void() &&>> callbacks;
|
|
callbacks_.swap(callbacks);
|
|
for (auto& callback : callbacks)
|
|
std::move(callback)();
|
|
},
|
|
tick_period_);
|
|
}
|
|
}
|
|
|
|
TimeDelta TaskQueueMetronome::TickPeriod() const {
|
|
return tick_period_;
|
|
}
|
|
|
|
} // namespace webrtc
|