mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 22:00:47 +01:00
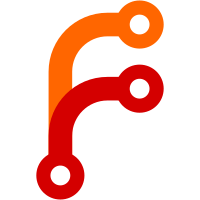
This member of the CodecInfo struct was set in several places, but not used for anything. To aid deletion, this cl defines a default implementation of VideoEncoderFactory::QueryVideoEncoder. The next step is to delete almost all downstream implementations of that method, since the only classes that have to implement it are the few factories that produce "internal source" encoders, e.g., for Chromium remoting. Bug: None Change-Id: I1f0dbf0d302933004ebdc779460cb2cb3a894e02 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/179520 Reviewed-by: Kári Helgason <kthelgason@webrtc.org> Reviewed-by: Sami Kalliomäki <sakal@webrtc.org> Reviewed-by: Sebastian Jansson <srte@webrtc.org> Commit-Queue: Niels Moller <nisse@webrtc.org> Cr-Commit-Position: refs/heads/master@{#31844}
60 lines
2.3 KiB
C++
60 lines
2.3 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "media/engine/internal_encoder_factory.h"
|
|
|
|
#include <string>
|
|
|
|
#include "absl/strings/match.h"
|
|
#include "api/video_codecs/sdp_video_format.h"
|
|
#include "media/base/codec.h"
|
|
#include "media/base/media_constants.h"
|
|
#include "modules/video_coding/codecs/av1/libaom_av1_encoder.h"
|
|
#include "modules/video_coding/codecs/h264/include/h264.h"
|
|
#include "modules/video_coding/codecs/vp8/include/vp8.h"
|
|
#include "modules/video_coding/codecs/vp9/include/vp9.h"
|
|
#include "rtc_base/logging.h"
|
|
|
|
namespace webrtc {
|
|
|
|
std::vector<SdpVideoFormat> InternalEncoderFactory::SupportedFormats() {
|
|
std::vector<SdpVideoFormat> supported_codecs;
|
|
supported_codecs.push_back(SdpVideoFormat(cricket::kVp8CodecName));
|
|
for (const webrtc::SdpVideoFormat& format : webrtc::SupportedVP9Codecs())
|
|
supported_codecs.push_back(format);
|
|
for (const webrtc::SdpVideoFormat& format : webrtc::SupportedH264Codecs())
|
|
supported_codecs.push_back(format);
|
|
if (kIsLibaomAv1EncoderSupported)
|
|
supported_codecs.push_back(SdpVideoFormat(cricket::kAv1CodecName));
|
|
return supported_codecs;
|
|
}
|
|
|
|
std::vector<SdpVideoFormat> InternalEncoderFactory::GetSupportedFormats()
|
|
const {
|
|
return SupportedFormats();
|
|
}
|
|
|
|
std::unique_ptr<VideoEncoder> InternalEncoderFactory::CreateVideoEncoder(
|
|
const SdpVideoFormat& format) {
|
|
if (absl::EqualsIgnoreCase(format.name, cricket::kVp8CodecName))
|
|
return VP8Encoder::Create();
|
|
if (absl::EqualsIgnoreCase(format.name, cricket::kVp9CodecName))
|
|
return VP9Encoder::Create(cricket::VideoCodec(format));
|
|
if (absl::EqualsIgnoreCase(format.name, cricket::kH264CodecName))
|
|
return H264Encoder::Create(cricket::VideoCodec(format));
|
|
if (kIsLibaomAv1EncoderSupported &&
|
|
absl::EqualsIgnoreCase(format.name, cricket::kAv1CodecName))
|
|
return CreateLibaomAv1Encoder();
|
|
RTC_LOG(LS_ERROR) << "Trying to created encoder of unsupported format "
|
|
<< format.name;
|
|
return nullptr;
|
|
}
|
|
|
|
} // namespace webrtc
|