mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 06:10:40 +01:00
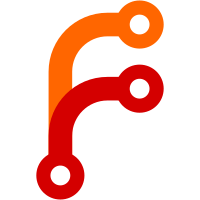
This patch adds (optional) csrc to ContributingSources. This will be used if using virtual audio ssrc, since the audio level is otherwise unaccessible in that configuration. BUG=webrtc:3333 Change-Id: Ied263b8f0850553cd637fd6bead373ed4252fd1e Reviewed-on: https://webrtc-review.googlesource.com/c/109281 Reviewed-by: Oskar Sundbom <ossu@webrtc.org> Reviewed-by: Åsa Persson <asapersson@webrtc.org> Reviewed-by: Niels Moller <nisse@webrtc.org> Reviewed-by: Danil Chapovalov <danilchap@webrtc.org> Commit-Queue: Jonas Oreland <jonaso@webrtc.org> Cr-Commit-Position: refs/heads/master@{#25516}
82 lines
2.7 KiB
C++
82 lines
2.7 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "modules/rtp_rtcp/source/contributing_sources.h"
|
|
|
|
namespace webrtc {
|
|
|
|
namespace {
|
|
|
|
// Allow some stale records to accumulate before cleaning.
|
|
constexpr int64_t kPruningIntervalMs = 15 * rtc::kNumMillisecsPerSec;
|
|
|
|
} // namespace
|
|
|
|
constexpr int64_t ContributingSources::kHistoryMs;
|
|
|
|
ContributingSources::ContributingSources() = default;
|
|
ContributingSources::~ContributingSources() = default;
|
|
|
|
void ContributingSources::Update(int64_t now_ms,
|
|
rtc::ArrayView<const uint32_t> csrcs,
|
|
absl::optional<uint8_t> audio_level) {
|
|
Entry entry = { now_ms, audio_level };
|
|
for (uint32_t csrc : csrcs) {
|
|
active_csrcs_[csrc] = entry;
|
|
}
|
|
if (!next_pruning_ms_) {
|
|
next_pruning_ms_ = now_ms + kPruningIntervalMs;
|
|
} else if (now_ms > next_pruning_ms_) {
|
|
// To prevent unlimited growth, prune it every 15 seconds.
|
|
DeleteOldEntries(now_ms);
|
|
}
|
|
}
|
|
|
|
// Return contributing sources seen the last 10 s.
|
|
// TODO(nisse): It would be more efficient to delete any stale entries while
|
|
// iterating over the mapping, but then we'd have to make the method
|
|
// non-const.
|
|
std::vector<RtpSource> ContributingSources::GetSources(int64_t now_ms) const {
|
|
std::vector<RtpSource> sources;
|
|
for (auto& record : active_csrcs_) {
|
|
if (record.second.last_seen_ms >= now_ms - kHistoryMs) {
|
|
if (record.second.audio_level.has_value()) {
|
|
sources.emplace_back(record.second.last_seen_ms, record.first,
|
|
RtpSourceType::CSRC,
|
|
*record.second.audio_level);
|
|
} else {
|
|
sources.emplace_back(record.second.last_seen_ms, record.first,
|
|
RtpSourceType::CSRC);
|
|
}
|
|
}
|
|
}
|
|
|
|
return sources;
|
|
}
|
|
|
|
// Delete stale entries.
|
|
void ContributingSources::DeleteOldEntries(int64_t now_ms) {
|
|
for (auto it = active_csrcs_.begin(); it != active_csrcs_.end();) {
|
|
if (it->second.last_seen_ms >= now_ms - kHistoryMs) {
|
|
// Still relevant.
|
|
++it;
|
|
} else {
|
|
it = active_csrcs_.erase(it);
|
|
}
|
|
}
|
|
next_pruning_ms_ = now_ms + kPruningIntervalMs;
|
|
}
|
|
|
|
ContributingSources::Entry::Entry() = default;
|
|
ContributingSources::Entry::Entry(int64_t timestamp_ms,
|
|
absl::optional<uint8_t> audio_level_arg)
|
|
: last_seen_ms(timestamp_ms), audio_level(audio_level_arg) {}
|
|
|
|
} // namespace webrtc
|