mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
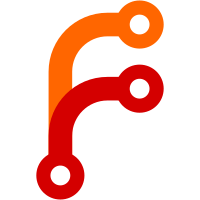
The 'Module' part of the implementation must not be called via the RtpRtcp interface, but is rather a part of the contract with ProcessThread. That in turn is an implementation detail for how timers are currently implemented in the default implementation. Along the way I'm deprecating away the factory function which was inside the interface and tied it to one specific implementation. Instead, I'm moving that to the implementation itself and down the line, we don't have to go through it if we just want to create an instance of the class. The key change is in rtp_rtcp.h and the new rtp_rtcp_interface.h header file (things moved from rtp_rtcp.h), the rest falls from that. Change-Id: I294f13e947b9e3e4e649400ee94a11a81e8071ce Bug: webrtc:11581 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/176419 Reviewed-by: Magnus Flodman <mflodman@webrtc.org> Commit-Queue: Tommi <tommi@webrtc.org> Cr-Commit-Position: refs/heads/master@{#31440}
67 lines
1.9 KiB
C++
67 lines
1.9 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef CALL_FLEXFEC_RECEIVE_STREAM_IMPL_H_
|
|
#define CALL_FLEXFEC_RECEIVE_STREAM_IMPL_H_
|
|
|
|
#include <memory>
|
|
|
|
#include "call/flexfec_receive_stream.h"
|
|
#include "call/rtp_packet_sink_interface.h"
|
|
#include "modules/rtp_rtcp/source/rtp_rtcp_impl2.h"
|
|
#include "system_wrappers/include/clock.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class FlexfecReceiver;
|
|
class ProcessThread;
|
|
class ReceiveStatistics;
|
|
class RecoveredPacketReceiver;
|
|
class RtcpRttStats;
|
|
class RtpPacketReceived;
|
|
class RtpRtcp;
|
|
class RtpStreamReceiverControllerInterface;
|
|
class RtpStreamReceiverInterface;
|
|
|
|
class FlexfecReceiveStreamImpl : public FlexfecReceiveStream {
|
|
public:
|
|
FlexfecReceiveStreamImpl(
|
|
Clock* clock,
|
|
RtpStreamReceiverControllerInterface* receiver_controller,
|
|
const Config& config,
|
|
RecoveredPacketReceiver* recovered_packet_receiver,
|
|
RtcpRttStats* rtt_stats,
|
|
ProcessThread* process_thread);
|
|
~FlexfecReceiveStreamImpl() override;
|
|
|
|
// RtpPacketSinkInterface.
|
|
void OnRtpPacket(const RtpPacketReceived& packet) override;
|
|
|
|
Stats GetStats() const override;
|
|
const Config& GetConfig() const override;
|
|
|
|
private:
|
|
// Config.
|
|
const Config config_;
|
|
|
|
// Erasure code interfacing.
|
|
const std::unique_ptr<FlexfecReceiver> receiver_;
|
|
|
|
// RTCP reporting.
|
|
const std::unique_ptr<ReceiveStatistics> rtp_receive_statistics_;
|
|
const std::unique_ptr<ModuleRtpRtcpImpl2> rtp_rtcp_;
|
|
ProcessThread* process_thread_;
|
|
|
|
std::unique_ptr<RtpStreamReceiverInterface> rtp_stream_receiver_;
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // CALL_FLEXFEC_RECEIVE_STREAM_IMPL_H_
|