mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 13:50:40 +01:00
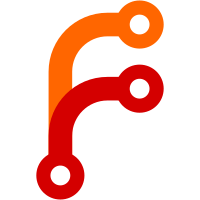
This CL extends the WebRTC testing API to allow audioproc_f -based testing using a pre-created AudioProcessing object. This is an important feature to allow testing any AudioProcessing objects that are injected into WebRTC. Beyond adding this, the CL also changes the simulation code to operate on a scoped_refptr<AudioProcessing> object instead of a std::unique<AudioProcessing> object Bug: webrtc:5298 Change-Id: I70179f19518fc583ad0101bd59c038478a3cc23d Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/175568 Commit-Queue: Per Åhgren <peah@webrtc.org> Reviewed-by: Karl Wiberg <kwiberg@webrtc.org> Reviewed-by: Sam Zackrisson <saza@webrtc.org> Cr-Commit-Position: refs/heads/master@{#31319}
74 lines
2.7 KiB
C++
74 lines
2.7 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_AUDIO_PROCESSING_TEST_AEC_DUMP_BASED_SIMULATOR_H_
|
|
#define MODULES_AUDIO_PROCESSING_TEST_AEC_DUMP_BASED_SIMULATOR_H_
|
|
|
|
#include <fstream>
|
|
#include <string>
|
|
|
|
#include "modules/audio_processing/test/audio_processing_simulator.h"
|
|
#include "rtc_base/constructor_magic.h"
|
|
#include "rtc_base/ignore_wundef.h"
|
|
|
|
RTC_PUSH_IGNORING_WUNDEF()
|
|
#ifdef WEBRTC_ANDROID_PLATFORM_BUILD
|
|
#include "external/webrtc/webrtc/modules/audio_processing/debug.pb.h"
|
|
#else
|
|
#include "modules/audio_processing/debug.pb.h"
|
|
#endif
|
|
RTC_POP_IGNORING_WUNDEF()
|
|
|
|
namespace webrtc {
|
|
namespace test {
|
|
|
|
// Used to perform an audio processing simulation from an aec dump.
|
|
class AecDumpBasedSimulator final : public AudioProcessingSimulator {
|
|
public:
|
|
AecDumpBasedSimulator(const SimulationSettings& settings,
|
|
rtc::scoped_refptr<AudioProcessing> audio_processing,
|
|
std::unique_ptr<AudioProcessingBuilder> ap_builder);
|
|
~AecDumpBasedSimulator() override;
|
|
|
|
// Processes the messages in the aecdump file.
|
|
void Process() override;
|
|
|
|
private:
|
|
void HandleEvent(const webrtc::audioproc::Event& event_msg,
|
|
int* num_forward_chunks_processed);
|
|
void HandleMessage(const webrtc::audioproc::Init& msg);
|
|
void HandleMessage(const webrtc::audioproc::Stream& msg);
|
|
void HandleMessage(const webrtc::audioproc::ReverseStream& msg);
|
|
void HandleMessage(const webrtc::audioproc::Config& msg);
|
|
void HandleMessage(const webrtc::audioproc::RuntimeSetting& msg);
|
|
void PrepareProcessStreamCall(const webrtc::audioproc::Stream& msg);
|
|
void PrepareReverseProcessStreamCall(
|
|
const webrtc::audioproc::ReverseStream& msg);
|
|
void VerifyProcessStreamBitExactness(const webrtc::audioproc::Stream& msg);
|
|
void MaybeOpenCallOrderFile();
|
|
enum InterfaceType {
|
|
kFixedInterface,
|
|
kFloatInterface,
|
|
kNotSpecified,
|
|
};
|
|
|
|
FILE* dump_input_file_;
|
|
std::unique_ptr<ChannelBuffer<float>> artificial_nearend_buf_;
|
|
std::unique_ptr<ChannelBufferWavReader> artificial_nearend_buffer_reader_;
|
|
bool artificial_nearend_eof_reported_ = false;
|
|
InterfaceType interface_used_ = InterfaceType::kNotSpecified;
|
|
std::unique_ptr<std::ofstream> call_order_output_file_;
|
|
RTC_DISALLOW_IMPLICIT_CONSTRUCTORS(AecDumpBasedSimulator);
|
|
};
|
|
|
|
} // namespace test
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_AUDIO_PROCESSING_TEST_AEC_DUMP_BASED_SIMULATOR_H_
|