mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-17 15:47:53 +01:00
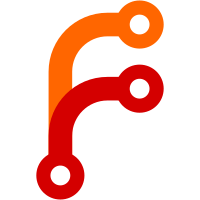
Reason for reland:
Added disabling of the BlockProcessor DEATH tests due to false alarms on the trybots.
These errors cannot be reproduced locally and they occur only in the DEATH test code,
which means that the memory leaks do not occur under normal conditions.
Original issue's description:
> Reason for revert:
> Memcheck buildbot detected memory leaks in the death tests. The code looks fine
> to me, but please investigate what causes the error and reland.
> > Original issue's description:
> > Added first layer of the echo canceller 3 functionality.
> >
> > This CL adds the first layer of the echo canceller 3.
> > All of the code is as it should, apart from
> > block_processor.* which only contains placeholder
> > functionality. (Upcoming CLs will add proper
> > functionality into those files.)
> >
> >
> >
> > BUG=webrtc:6018
> >
> > Review-Url: https://codereview.webrtc.org/2584493002
> > Cr-Commit-Position: refs/heads/master@{#15861}
> > Committed:
> 38fd1758e9
>
> TBR=ivoc@webrtc.org,aleloi@webrtc.org,henrik.lundin@webrtc.org,peah@webrtc.org
> # Skipping CQ checks because original CL landed less than 1 days ago.
> NOPRESUBMIT=true
> NOTREECHECKS=true
> NOTRY=true
> BUG=webrtc:6018
BUG=webrtc:6018
TBR=ivoc@webrtc.org,aleloi@webrtc.org,henrik.lundin@webrtc.org
Review-Url: https://codereview.webrtc.org/2608233002
Cr-Commit-Position: refs/heads/master@{#15884}
73 lines
2.4 KiB
C++
73 lines
2.4 KiB
C++
/*
|
|
* Copyright (c) 2016 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#include "webrtc/modules/audio_processing/aec3/block_processor.h"
|
|
|
|
#include "webrtc/base/atomicops.h"
|
|
#include "webrtc/base/optional.h"
|
|
#include "webrtc/modules/audio_processing/aec3/aec3_constants.h"
|
|
|
|
namespace webrtc {
|
|
namespace {
|
|
|
|
class BlockProcessorImpl final : public BlockProcessor {
|
|
public:
|
|
explicit BlockProcessorImpl(int sample_rate_hz);
|
|
~BlockProcessorImpl() override;
|
|
|
|
void ProcessCapture(bool known_echo_path_change,
|
|
bool saturated_microphone_signal,
|
|
std::vector<std::vector<float>>* capture_block) override;
|
|
|
|
bool BufferRender(std::vector<std::vector<float>>* block) override;
|
|
|
|
void ReportEchoLeakage(bool leakage_detected) override;
|
|
|
|
private:
|
|
const size_t sample_rate_hz_;
|
|
static int instance_count_;
|
|
std::unique_ptr<ApmDataDumper> data_dumper_;
|
|
RTC_DISALLOW_IMPLICIT_CONSTRUCTORS(BlockProcessorImpl);
|
|
};
|
|
|
|
int BlockProcessorImpl::instance_count_ = 0;
|
|
|
|
BlockProcessorImpl::BlockProcessorImpl(int sample_rate_hz)
|
|
: sample_rate_hz_(sample_rate_hz),
|
|
data_dumper_(
|
|
new ApmDataDumper(rtc::AtomicOps::Increment(&instance_count_))) {}
|
|
|
|
BlockProcessorImpl::~BlockProcessorImpl() = default;
|
|
|
|
void BlockProcessorImpl::ProcessCapture(
|
|
bool known_echo_path_change,
|
|
bool saturated_microphone_signal,
|
|
std::vector<std::vector<float>>* capture_block) {
|
|
RTC_DCHECK(capture_block);
|
|
RTC_DCHECK_EQ(NumBandsForRate(sample_rate_hz_), capture_block->size());
|
|
RTC_DCHECK_EQ(kBlockSize, (*capture_block)[0].size());
|
|
}
|
|
|
|
bool BlockProcessorImpl::BufferRender(
|
|
std::vector<std::vector<float>>* render_block) {
|
|
RTC_DCHECK(render_block);
|
|
RTC_DCHECK_EQ(NumBandsForRate(sample_rate_hz_), render_block->size());
|
|
RTC_DCHECK_EQ(kBlockSize, (*render_block)[0].size());
|
|
return false;
|
|
}
|
|
|
|
void BlockProcessorImpl::ReportEchoLeakage(bool leakage_detected) {}
|
|
|
|
} // namespace
|
|
|
|
BlockProcessor* BlockProcessor::Create(int sample_rate_hz) {
|
|
return new BlockProcessorImpl(sample_rate_hz);
|
|
}
|
|
|
|
} // namespace webrtc
|