mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 06:10:40 +01:00
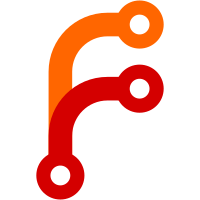
The number of audio channels can be configured in SDP, and can thus be set to arbitrary values. However, the audio code has limitations that prevent a high number of channels from working well in practice. Bug: chromium:1265806 Change-Id: I6f6c3f68a3791bb189a614eece6bd0ed7874f252 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/237807 Reviewed-by: Jakob Ivarsson <jakobi@webrtc.org> Reviewed-by: Harald Alvestrand <hta@webrtc.org> Commit-Queue: Ivo Creusen <ivoc@webrtc.org> Cr-Commit-Position: refs/heads/main@{#35359}
52 lines
1.8 KiB
C++
52 lines
1.8 KiB
C++
/*
|
|
* Copyright (c) 2017 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef API_AUDIO_CODECS_G711_AUDIO_ENCODER_G711_H_
|
|
#define API_AUDIO_CODECS_G711_AUDIO_ENCODER_G711_H_
|
|
|
|
#include <memory>
|
|
#include <vector>
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/audio_codecs/audio_codec_pair_id.h"
|
|
#include "api/audio_codecs/audio_encoder.h"
|
|
#include "api/audio_codecs/audio_format.h"
|
|
#include "rtc_base/system/rtc_export.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// G711 encoder API for use as a template parameter to
|
|
// CreateAudioEncoderFactory<...>().
|
|
struct RTC_EXPORT AudioEncoderG711 {
|
|
struct Config {
|
|
enum class Type { kPcmU, kPcmA };
|
|
bool IsOk() const {
|
|
return (type == Type::kPcmU || type == Type::kPcmA) &&
|
|
frame_size_ms > 0 && frame_size_ms % 10 == 0 &&
|
|
num_channels >= 1 &&
|
|
num_channels <= AudioEncoder::kMaxNumberOfChannels;
|
|
}
|
|
Type type = Type::kPcmU;
|
|
int num_channels = 1;
|
|
int frame_size_ms = 20;
|
|
};
|
|
static absl::optional<AudioEncoderG711::Config> SdpToConfig(
|
|
const SdpAudioFormat& audio_format);
|
|
static void AppendSupportedEncoders(std::vector<AudioCodecSpec>* specs);
|
|
static AudioCodecInfo QueryAudioEncoder(const Config& config);
|
|
static std::unique_ptr<AudioEncoder> MakeAudioEncoder(
|
|
const Config& config,
|
|
int payload_type,
|
|
absl::optional<AudioCodecPairId> codec_pair_id = absl::nullopt);
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // API_AUDIO_CODECS_G711_AUDIO_ENCODER_G711_H_
|