mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-13 05:40:42 +01:00
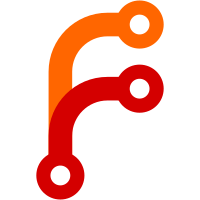
I gave up on removing proxy_info, user_agent and tcp_options. I don't think it's feasible to remove them without removing all the proxy code. The assumption that you can set the proxy and user agent long after you have created the factory is entrenched in unit tests and the code itself. So is the ability to set tcp opts depending on protocol or endpoint properties. It may be easier to untangle proxy stuff from the factory later, when it becomes a more first-class citizen and isn't passed via the allocator. Requires https://chromium-review.googlesource.com/c/chromium/src/+/1778870 to land first. Bug: webrtc:7447 Change-Id: Ib496e2bb689ea415e9f8ec1dfedff13a83fa4a8a Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/150799 Commit-Queue: Patrik Höglund <phoglund@webrtc.org> Reviewed-by: Niels Moller <nisse@webrtc.org> Cr-Commit-Position: refs/heads/master@{#29091}
81 lines
2.5 KiB
C++
81 lines
2.5 KiB
C++
/*
|
|
* Copyright 2019 The WebRTC Project Authors. All rights reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef API_PACKET_SOCKET_FACTORY_H_
|
|
#define API_PACKET_SOCKET_FACTORY_H_
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "rtc_base/async_packet_socket.h"
|
|
#include "rtc_base/proxy_info.h"
|
|
#include "rtc_base/system/rtc_export.h"
|
|
|
|
namespace rtc {
|
|
|
|
class SSLCertificateVerifier;
|
|
class AsyncResolverInterface;
|
|
|
|
struct PacketSocketTcpOptions {
|
|
PacketSocketTcpOptions() = default;
|
|
~PacketSocketTcpOptions() = default;
|
|
|
|
int opts = 0;
|
|
std::vector<std::string> tls_alpn_protocols;
|
|
std::vector<std::string> tls_elliptic_curves;
|
|
// An optional custom SSL certificate verifier that an API user can provide to
|
|
// inject their own certificate verification logic (not available to users
|
|
// outside of the WebRTC repo).
|
|
SSLCertificateVerifier* tls_cert_verifier = nullptr;
|
|
};
|
|
|
|
class RTC_EXPORT PacketSocketFactory {
|
|
public:
|
|
enum Options {
|
|
OPT_STUN = 0x04,
|
|
|
|
// The TLS options below are mutually exclusive.
|
|
OPT_TLS = 0x02, // Real and secure TLS.
|
|
OPT_TLS_FAKE = 0x01, // Fake TLS with a dummy SSL handshake.
|
|
OPT_TLS_INSECURE = 0x08, // Insecure TLS without certificate validation.
|
|
|
|
// Deprecated, use OPT_TLS_FAKE.
|
|
OPT_SSLTCP = OPT_TLS_FAKE,
|
|
};
|
|
|
|
PacketSocketFactory() = default;
|
|
virtual ~PacketSocketFactory() = default;
|
|
|
|
virtual AsyncPacketSocket* CreateUdpSocket(const SocketAddress& address,
|
|
uint16_t min_port,
|
|
uint16_t max_port) = 0;
|
|
virtual AsyncPacketSocket* CreateServerTcpSocket(
|
|
const SocketAddress& local_address,
|
|
uint16_t min_port,
|
|
uint16_t max_port,
|
|
int opts) = 0;
|
|
|
|
virtual AsyncPacketSocket* CreateClientTcpSocket(
|
|
const SocketAddress& local_address,
|
|
const SocketAddress& remote_address,
|
|
const ProxyInfo& proxy_info,
|
|
const std::string& user_agent,
|
|
const PacketSocketTcpOptions& tcp_options) = 0;
|
|
|
|
virtual AsyncResolverInterface* CreateAsyncResolver() = 0;
|
|
|
|
private:
|
|
PacketSocketFactory(const PacketSocketFactory&) = delete;
|
|
PacketSocketFactory& operator=(const PacketSocketFactory&) = delete;
|
|
};
|
|
|
|
} // namespace rtc
|
|
|
|
#endif // API_PACKET_SOCKET_FACTORY_H_
|