mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-15 06:40:43 +01:00
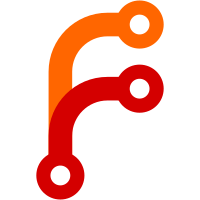
The previous name packet queue 2 had no indication on what the difference was compared to the regular packet queue. This rename makes it easier to understand the codebase. Additionally the PacketQueueInterface class was introduced to make the class hierarchy easier to follow. The round robin packet queue did not extend the packet queue so there was no reason for inheriting from the specific implementation. Bug: None Change-Id: Idbce081c751fbacd927632f5e71220887d0b5991 Reviewed-on: https://webrtc-review.googlesource.com/49120 Commit-Queue: Sebastian Jansson <srte@webrtc.org> Reviewed-by: Stefan Holmer <stefan@webrtc.org> Cr-Commit-Position: refs/heads/master@{#21931}
69 lines
2.2 KiB
C++
69 lines
2.2 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_PACING_PACKET_QUEUE_INTERFACE_H_
|
|
#define MODULES_PACING_PACKET_QUEUE_INTERFACE_H_
|
|
|
|
#include <stdint.h>
|
|
|
|
#include <list>
|
|
#include <queue>
|
|
#include <set>
|
|
|
|
#include "modules/rtp_rtcp/include/rtp_rtcp_defines.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class PacketQueueInterface {
|
|
public:
|
|
PacketQueueInterface() = default;
|
|
virtual ~PacketQueueInterface() = default;
|
|
|
|
struct Packet {
|
|
Packet(RtpPacketSender::Priority priority,
|
|
uint32_t ssrc,
|
|
uint16_t seq_number,
|
|
int64_t capture_time_ms,
|
|
int64_t enqueue_time_ms,
|
|
size_t length_in_bytes,
|
|
bool retransmission,
|
|
uint64_t enqueue_order);
|
|
Packet(const Packet& other);
|
|
virtual ~Packet();
|
|
bool operator<(const Packet& other) const;
|
|
|
|
RtpPacketSender::Priority priority;
|
|
uint32_t ssrc;
|
|
uint16_t sequence_number;
|
|
int64_t capture_time_ms; // Absolute time of frame capture.
|
|
int64_t enqueue_time_ms; // Absolute time of pacer queue entry.
|
|
int64_t sum_paused_ms;
|
|
size_t bytes;
|
|
bool retransmission;
|
|
uint64_t enqueue_order;
|
|
std::list<Packet>::iterator this_it;
|
|
std::multiset<int64_t>::iterator enqueue_time_it;
|
|
};
|
|
|
|
virtual void Push(const Packet& packet) = 0;
|
|
virtual const Packet& BeginPop() = 0;
|
|
virtual void CancelPop(const Packet& packet) = 0;
|
|
virtual void FinalizePop(const Packet& packet) = 0;
|
|
virtual bool Empty() const = 0;
|
|
virtual size_t SizeInPackets() const = 0;
|
|
virtual uint64_t SizeInBytes() const = 0;
|
|
virtual int64_t OldestEnqueueTimeMs() const = 0;
|
|
virtual void UpdateQueueTime(int64_t timestamp_ms) = 0;
|
|
virtual void SetPauseState(bool paused, int64_t timestamp_ms) = 0;
|
|
virtual int64_t AverageQueueTimeMs() const = 0;
|
|
};
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_PACING_PACKET_QUEUE_INTERFACE_H_
|