mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-16 23:30:48 +01:00
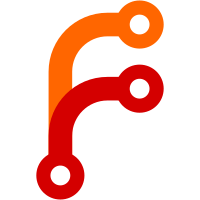
This changes the behavior for adding virtual transport overhead so it doesn't change the size of the actual payload buffer, only the calculated packet size. Bug: webrtc:9883 Change-Id: I6e24598378c4dd6a591d36ca3b162e933ff4ef7c Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/164523 Commit-Queue: Sebastian Jansson <srte@webrtc.org> Reviewed-by: Artem Titov <titovartem@webrtc.org> Cr-Commit-Position: refs/heads/master@{#30298}
36 lines
1.3 KiB
C++
36 lines
1.3 KiB
C++
/*
|
|
* Copyright (c) 2019 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
#include "api/test/network_emulation/network_emulation_interfaces.h"
|
|
|
|
namespace webrtc {
|
|
|
|
namespace {
|
|
constexpr int kIPv4HeaderSize = 20;
|
|
constexpr int kIPv6HeaderSize = 40;
|
|
constexpr int kUdpHeaderSize = 8;
|
|
int IpHeaderSize(const rtc::SocketAddress& address) {
|
|
return (address.family() == AF_INET) ? kIPv4HeaderSize : kIPv6HeaderSize;
|
|
}
|
|
} // namespace
|
|
|
|
EmulatedIpPacket::EmulatedIpPacket(const rtc::SocketAddress& from,
|
|
const rtc::SocketAddress& to,
|
|
rtc::CopyOnWriteBuffer data,
|
|
Timestamp arrival_time,
|
|
uint16_t application_overhead)
|
|
: from(from),
|
|
to(to),
|
|
data(data),
|
|
headers_size(IpHeaderSize(to) + application_overhead + kUdpHeaderSize),
|
|
arrival_time(arrival_time) {
|
|
RTC_DCHECK(to.family() == AF_INET || to.family() == AF_INET6);
|
|
}
|
|
|
|
} // namespace webrtc
|