mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 14:20:45 +01:00
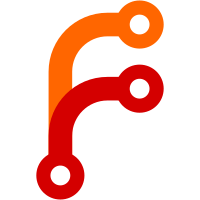
This is a reland of 18c4261339
Original change's description:
> Enables/disables simulcast streams by allocating a bitrate of 0 to the spatial layer.
>
> Creates VideoStreams & VideoCodec.simulcastStreams with an active field, and then allocates 0 bitrate to simulcast streams that are inactive. This turns off the encoder for specific simulcast streams.
>
> Bug: webrtc:8653
> Change-Id: Id93b03dcd8d1191a7d3300bd77882c8af96ee469
> Reviewed-on: https://webrtc-review.googlesource.com/37740
> Reviewed-by: Stefan Holmer <stefan@webrtc.org>
> Reviewed-by: Taylor Brandstetter <deadbeef@webrtc.org>
> Reviewed-by: Erik Språng <sprang@webrtc.org>
> Commit-Queue: Seth Hampson <shampson@webrtc.org>
> Cr-Commit-Position: refs/heads/master@{#21646}
TBR=sprang@webrtc.org,stefan@webrtc.org,deadbeef@webrtc.org
Bug: webrtc:8630
Change-Id: Ib3df6f9b7158bff362a7ec66fc57e368682c5846
Reviewed-on: https://webrtc-review.googlesource.com/40980
Reviewed-by: Seth Hampson <shampson@webrtc.org>
Reviewed-by: Erik Språng <sprang@webrtc.org>
Commit-Queue: Seth Hampson <shampson@webrtc.org>
Cr-Commit-Position: refs/heads/master@{#21688}
82 lines
2.2 KiB
C++
82 lines
2.2 KiB
C++
/*
|
|
* Copyright (c) 2014 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include "modules/video_coding/codecs/vp8/simulcast_test_utility.h"
|
|
|
|
namespace webrtc {
|
|
namespace testing {
|
|
|
|
class TestVp8Impl : public TestVp8Simulcast {
|
|
protected:
|
|
std::unique_ptr<VP8Encoder> CreateEncoder() override {
|
|
return VP8Encoder::Create();
|
|
}
|
|
std::unique_ptr<VP8Decoder> CreateDecoder() override {
|
|
return VP8Decoder::Create();
|
|
}
|
|
};
|
|
|
|
TEST_F(TestVp8Impl, TestKeyFrameRequestsOnAllStreams) {
|
|
TestVp8Simulcast::TestKeyFrameRequestsOnAllStreams();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestPaddingAllStreams) {
|
|
TestVp8Simulcast::TestPaddingAllStreams();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestPaddingTwoStreams) {
|
|
TestVp8Simulcast::TestPaddingTwoStreams();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestPaddingTwoStreamsOneMaxedOut) {
|
|
TestVp8Simulcast::TestPaddingTwoStreamsOneMaxedOut();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestPaddingOneStream) {
|
|
TestVp8Simulcast::TestPaddingOneStream();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestPaddingOneStreamTwoMaxedOut) {
|
|
TestVp8Simulcast::TestPaddingOneStreamTwoMaxedOut();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestSendAllStreams) {
|
|
TestVp8Simulcast::TestSendAllStreams();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestDisablingStreams) {
|
|
TestVp8Simulcast::TestDisablingStreams();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestActiveStreams) {
|
|
TestVp8Simulcast::TestActiveStreams();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestSwitchingToOneStream) {
|
|
TestVp8Simulcast::TestSwitchingToOneStream();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestSwitchingToOneOddStream) {
|
|
TestVp8Simulcast::TestSwitchingToOneOddStream();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestSwitchingToOneSmallStream) {
|
|
TestVp8Simulcast::TestSwitchingToOneSmallStream();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestSaptioTemporalLayers333PatternEncoder) {
|
|
TestVp8Simulcast::TestSaptioTemporalLayers333PatternEncoder();
|
|
}
|
|
|
|
TEST_F(TestVp8Impl, TestStrideEncodeDecode) {
|
|
TestVp8Simulcast::TestStrideEncodeDecode();
|
|
}
|
|
} // namespace testing
|
|
} // namespace webrtc
|