mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-15 14:50:39 +01:00
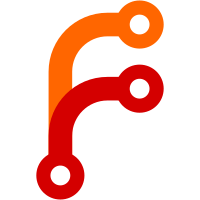
PeerConnection now has a new setting in RTCConfiguration to enable use of datagram transport for data channels. There is also a corresponding field trial, which has both a kill-switch and a way to change the default value. PeerConnection's interaction with MediaTransport for data channels has been refactored to work with DataChannelTransportInterface instead. Adds a DataChannelState and OnStateChanged() to the DataChannelSink callbacks. This allows PeerConnection to listen to the data channel's state directly, instead of indirectly by monitoring media transport state. This is necessary to enable use of non-media-transport (eg. datagram transport) data channel transports. For now, PeerConnection watches the state through MediaTransport as well. This will persist until MediaTransport implements the new callback. Datagram transport use is negotiated. As such, an offer that requests to use datagram transport for data channels may be rejected by the answerer. If the offer includes DTLS, the data channels will be negotiated as SCTP/DTLS data channels with an extra x-opaque parameter for datagram transport. If the opaque parameter is rejected (by an answerer without datagram support), the offerer may fall back to SCTP. If DTLS is not enabled, there is no viable fallback. In this case, the data channels are negotiated as media transport data channels. If the receiver does not understand the x-opaque line, it will reject these data channels, and the offerer's data channels will be closed. Bug: webrtc:9719 Change-Id: Ic1bf3664c4bcf9d754482df59897f5f72fe68fcc Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/147702 Commit-Queue: Bjorn Mellem <mellem@webrtc.org> Reviewed-by: Steve Anton <steveanton@webrtc.org> Cr-Commit-Position: refs/heads/master@{#28932}
125 lines
4.9 KiB
C++
125 lines
4.9 KiB
C++
/* Copyright 2019 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
// This is an experimental interface and is subject to change without notice.
|
|
|
|
#ifndef API_DATA_CHANNEL_TRANSPORT_INTERFACE_H_
|
|
#define API_DATA_CHANNEL_TRANSPORT_INTERFACE_H_
|
|
|
|
#include "absl/types/optional.h"
|
|
#include "api/rtc_error.h"
|
|
#include "rtc_base/copy_on_write_buffer.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// Supported types of application data messages.
|
|
enum class DataMessageType {
|
|
// Application data buffer with the binary bit unset.
|
|
kText,
|
|
|
|
// Application data buffer with the binary bit set.
|
|
kBinary,
|
|
|
|
// Transport-agnostic control messages, such as open or open-ack messages.
|
|
kControl,
|
|
};
|
|
|
|
// Parameters for sending data. The parameters may change from message to
|
|
// message, even within a single channel. For example, control messages may be
|
|
// sent reliably and in-order, even if the data channel is configured for
|
|
// unreliable delivery.
|
|
struct SendDataParams {
|
|
SendDataParams();
|
|
SendDataParams(const SendDataParams&);
|
|
|
|
DataMessageType type = DataMessageType::kText;
|
|
|
|
// Whether to deliver the message in order with respect to other ordered
|
|
// messages with the same channel_id.
|
|
bool ordered = false;
|
|
|
|
// If set, the maximum number of times this message may be
|
|
// retransmitted by the transport before it is dropped.
|
|
// Setting this value to zero disables retransmission.
|
|
// Must be non-negative. |max_rtx_count| and |max_rtx_ms| may not be set
|
|
// simultaneously.
|
|
absl::optional<int> max_rtx_count;
|
|
|
|
// If set, the maximum number of milliseconds for which the transport
|
|
// may retransmit this message before it is dropped.
|
|
// Setting this value to zero disables retransmission.
|
|
// Must be non-negative. |max_rtx_count| and |max_rtx_ms| may not be set
|
|
// simultaneously.
|
|
absl::optional<int> max_rtx_ms;
|
|
};
|
|
|
|
// Sink for callbacks related to a data channel.
|
|
class DataChannelSink {
|
|
public:
|
|
virtual ~DataChannelSink() = default;
|
|
|
|
// Callback issued when data is received by the transport.
|
|
virtual void OnDataReceived(int channel_id,
|
|
DataMessageType type,
|
|
const rtc::CopyOnWriteBuffer& buffer) = 0;
|
|
|
|
// Callback issued when a remote data channel begins the closing procedure.
|
|
// Messages sent after the closing procedure begins will not be transmitted.
|
|
virtual void OnChannelClosing(int channel_id) = 0;
|
|
|
|
// Callback issued when a (remote or local) data channel completes the closing
|
|
// procedure. Closing channels become closed after all pending data has been
|
|
// transmitted.
|
|
virtual void OnChannelClosed(int channel_id) = 0;
|
|
|
|
// Callback issued when the data channel becomes ready to send.
|
|
// This callback will be issued immediately when the data channel sink is
|
|
// registered if the transport is ready at that time. This callback may be
|
|
// invoked again following send errors (eg. due to the transport being
|
|
// temporarily blocked or unavailable).
|
|
// TODO(mellem): Make pure virtual when downstream sinks override this.
|
|
virtual void OnReadyToSend();
|
|
};
|
|
|
|
// Transport for data channels.
|
|
class DataChannelTransportInterface {
|
|
public:
|
|
virtual ~DataChannelTransportInterface() = default;
|
|
|
|
// Opens a data |channel_id| for sending. May return an error if the
|
|
// specified |channel_id| is unusable. Must be called before |SendData|.
|
|
virtual RTCError OpenChannel(int channel_id);
|
|
|
|
// Sends a data buffer to the remote endpoint using the given send parameters.
|
|
// |buffer| may not be larger than 256 KiB. Returns an error if the send
|
|
// fails.
|
|
virtual RTCError SendData(int channel_id,
|
|
const SendDataParams& params,
|
|
const rtc::CopyOnWriteBuffer& buffer);
|
|
|
|
// Closes |channel_id| gracefully. Returns an error if |channel_id| is not
|
|
// open. Data sent after the closing procedure begins will not be
|
|
// transmitted. The channel becomes closed after pending data is transmitted.
|
|
virtual RTCError CloseChannel(int channel_id);
|
|
|
|
// Sets a sink for data messages and channel state callbacks. Before media
|
|
// transport is destroyed, the sink must be unregistered by setting it to
|
|
// nullptr.
|
|
virtual void SetDataSink(DataChannelSink* sink);
|
|
|
|
// Returns whether this data channel transport is ready to send.
|
|
// Note: the default implementation always returns false (as it assumes no one
|
|
// has implemented the interface). This default implementation is temporary.
|
|
// TODO(mellem): Change this to pure virtual.
|
|
virtual bool IsReadyToSend() const;
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // API_DATA_CHANNEL_TRANSPORT_INTERFACE_H_
|