mirror of
https://github.com/mollyim/webrtc.git
synced 2025-05-14 06:10:40 +01:00
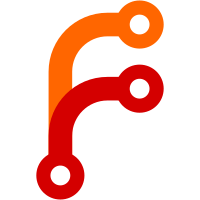
The dominant nearend detector uses the residual echo spectrum for determining whether in nearend state. The residual echo spectrum in computed using the ERLE. To reduce the risk of echo leaks in the suppressor, the ERLE is capped. While minimizing echo leaks, the capping of the ERLE can affect the dominant nearend classification negatively as the residual echo spectrum is often over estimated. This change enables the dominant nearend detector to use a residual echo spectrum computed with a virtually non-capped ERLE. This ERLE is only used for dominant nearend detection and leads to increased transparency. The feature is currently disabled by default and can be enabled with the field trial "WebRTC-Aec3UseUnboundedEchoSpectrum". Bug: webrtc:12870 Change-Id: Icb675c6f5d42ab9286e623b5fb38424d5c9cbee4 Reviewed-on: https://webrtc-review.googlesource.com/c/src/+/221920 Reviewed-by: Jesus de Vicente Pena <devicentepena@webrtc.org> Commit-Queue: Gustaf Ullberg <gustaf@webrtc.org> Cr-Commit-Position: refs/heads/master@{#34270}
106 lines
3.9 KiB
C++
106 lines
3.9 KiB
C++
/*
|
|
* Copyright (c) 2018 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef MODULES_AUDIO_PROCESSING_AEC3_SUBBAND_ERLE_ESTIMATOR_H_
|
|
#define MODULES_AUDIO_PROCESSING_AEC3_SUBBAND_ERLE_ESTIMATOR_H_
|
|
|
|
#include <stddef.h>
|
|
|
|
#include <array>
|
|
#include <memory>
|
|
#include <vector>
|
|
|
|
#include "api/array_view.h"
|
|
#include "api/audio/echo_canceller3_config.h"
|
|
#include "modules/audio_processing/aec3/aec3_common.h"
|
|
#include "modules/audio_processing/logging/apm_data_dumper.h"
|
|
|
|
namespace webrtc {
|
|
|
|
// Estimates the echo return loss enhancement for each frequency subband.
|
|
class SubbandErleEstimator {
|
|
public:
|
|
SubbandErleEstimator(const EchoCanceller3Config& config,
|
|
size_t num_capture_channels);
|
|
~SubbandErleEstimator();
|
|
|
|
// Resets the ERLE estimator.
|
|
void Reset();
|
|
|
|
// Updates the ERLE estimate.
|
|
void Update(rtc::ArrayView<const float, kFftLengthBy2Plus1> X2,
|
|
rtc::ArrayView<const std::array<float, kFftLengthBy2Plus1>> Y2,
|
|
rtc::ArrayView<const std::array<float, kFftLengthBy2Plus1>> E2,
|
|
const std::vector<bool>& converged_filters);
|
|
|
|
// Returns the ERLE estimate.
|
|
rtc::ArrayView<const std::array<float, kFftLengthBy2Plus1>> Erle(
|
|
bool onset_compensated) const {
|
|
return onset_compensated && use_onset_detection_ ? erle_onset_compensated_
|
|
: erle_;
|
|
}
|
|
|
|
// Returns the non-capped ERLE estimate.
|
|
rtc::ArrayView<const std::array<float, kFftLengthBy2Plus1>> ErleUnbounded()
|
|
const {
|
|
return erle_unbounded_;
|
|
}
|
|
|
|
// Returns the ERLE estimate at onsets (only used for testing).
|
|
rtc::ArrayView<const std::array<float, kFftLengthBy2Plus1>> ErleDuringOnsets()
|
|
const {
|
|
return erle_during_onsets_;
|
|
}
|
|
|
|
void Dump(const std::unique_ptr<ApmDataDumper>& data_dumper) const;
|
|
|
|
private:
|
|
struct AccumulatedSpectra {
|
|
explicit AccumulatedSpectra(size_t num_capture_channels)
|
|
: Y2(num_capture_channels),
|
|
E2(num_capture_channels),
|
|
low_render_energy(num_capture_channels),
|
|
num_points(num_capture_channels) {}
|
|
std::vector<std::array<float, kFftLengthBy2Plus1>> Y2;
|
|
std::vector<std::array<float, kFftLengthBy2Plus1>> E2;
|
|
std::vector<std::array<bool, kFftLengthBy2Plus1>> low_render_energy;
|
|
std::vector<int> num_points;
|
|
};
|
|
|
|
void UpdateAccumulatedSpectra(
|
|
rtc::ArrayView<const float, kFftLengthBy2Plus1> X2,
|
|
rtc::ArrayView<const std::array<float, kFftLengthBy2Plus1>> Y2,
|
|
rtc::ArrayView<const std::array<float, kFftLengthBy2Plus1>> E2,
|
|
const std::vector<bool>& converged_filters);
|
|
|
|
void ResetAccumulatedSpectra();
|
|
|
|
void UpdateBands(const std::vector<bool>& converged_filters);
|
|
void DecreaseErlePerBandForLowRenderSignals();
|
|
|
|
const bool use_onset_detection_;
|
|
const float min_erle_;
|
|
const std::array<float, kFftLengthBy2Plus1> max_erle_;
|
|
const bool use_min_erle_during_onsets_;
|
|
AccumulatedSpectra accum_spectra_;
|
|
// ERLE without special handling of render onsets.
|
|
std::vector<std::array<float, kFftLengthBy2Plus1>> erle_;
|
|
// ERLE lowered during render onsets.
|
|
std::vector<std::array<float, kFftLengthBy2Plus1>> erle_onset_compensated_;
|
|
std::vector<std::array<float, kFftLengthBy2Plus1>> erle_unbounded_;
|
|
// Estimation of ERLE during render onsets.
|
|
std::vector<std::array<float, kFftLengthBy2Plus1>> erle_during_onsets_;
|
|
std::vector<std::array<bool, kFftLengthBy2Plus1>> coming_onset_;
|
|
std::vector<std::array<int, kFftLengthBy2Plus1>> hold_counters_;
|
|
};
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // MODULES_AUDIO_PROCESSING_AEC3_SUBBAND_ERLE_ESTIMATOR_H_
|